Table Of Content
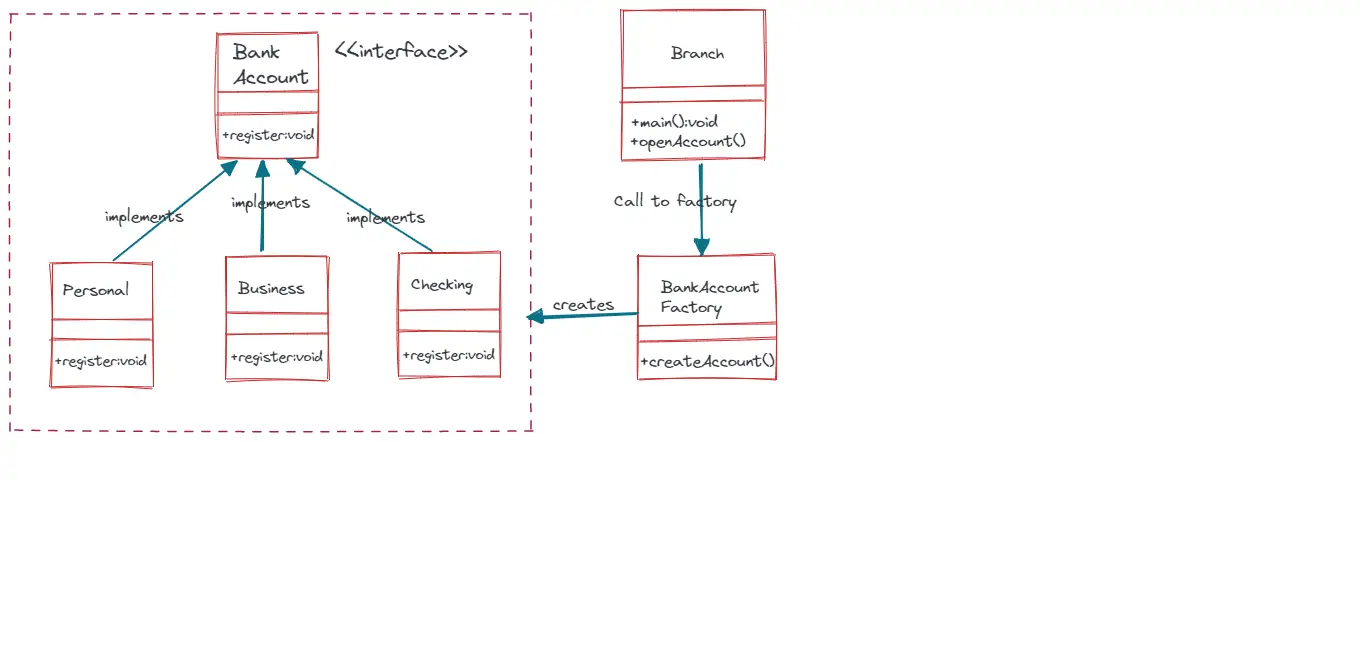
However, this will lead to the create(AccountType type) method becoming increasingly bloated. AccountFactory is a class that implements the Factory design pattern. It uses the accountType to create the corresponding type of BankAccount. This approach separates the object creation from the implementation, which promotes loose coupling and thus easier maintenance and upgrades.
How to Implement the Factory Design Pattern ❓
The current implementation of .get_serializer() is the same you used in the original example. The method evaluates the value of format and decides the concrete implementation to create and return. It is a relatively simple solution that allows us to verify the functionality of all the Factory Method components.
Zahner Factory Expansion – An Investigation on Patterns on Metal / Crawford Architects - eVolo
Zahner Factory Expansion – An Investigation on Patterns on Metal / Crawford Architects.
Posted: Tue, 27 Sep 2011 07:00:00 GMT [source]
Example implementations
For this reason, design patterns have become the de facto standard in the programming industry since their initial use a few decades ago due to their ability to solve many of these problems. In this article, we will dive deeper into one of these methodologies - namely, the Factory Method Pattern. As you can see, this class implements the CreditCard interface and provides implementation to all three methods. Similarly, we must do the same for the other two credit card classes. Here, we need to create either an interface or an abstract class that will expose the operations a credit card should have.
How ASML became Europe’s most valuable tech firm - BBC.com
How ASML became Europe’s most valuable tech firm.
Posted: Mon, 20 Feb 2023 08:00:00 GMT [source]
Solution
This logic will stay hidden from the end user, as we'll be specifying which implementation we want through the argument passed to the SpaceshipFactory method used for instantiation. Once the GetCreditCard method returns the appropriate product instance, we consume the methods as it is. Here, we are storing the object in the super interface GetCreditCard. Our factory class is responsible for creating and returning the appropriate Product (i.e., MoneyBack, Titanium, and Platinum) object. The config dictionary contains all the values required to initialize each of the services. The next step is to define an interface that will use those values to create a concrete implementation of a music service.
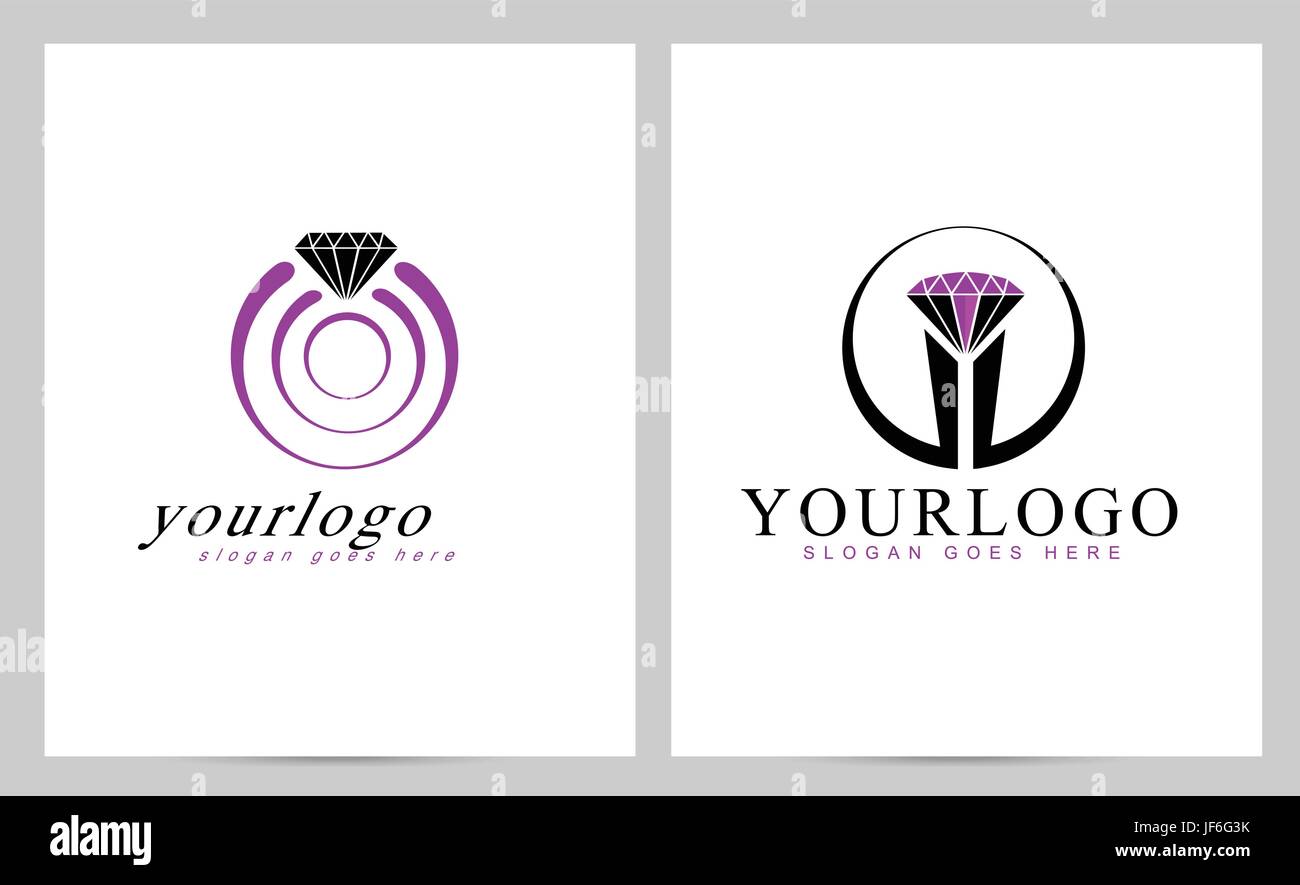
The single responsibility principle states that a module, a class, or even a method should have a single, well-defined responsibility. Use the Factory Method when you don’t know beforehand the exact types and dependencies of the objects your code should work with. Of course, you can apply this approach to other UI elements as well. However, with each new factory method you add to the Dialog, you get closer to the Abstract Factory pattern. Adding Ships into the app would require making changes to the entire codebase. Moreover, if later you decide to add another type of transportation to the app, you will probably need to make all of these changes again.
In this article, we will explore the Factory Design Pattern in detail and look at its various use cases and implementation techniques. Pankaj, You have a wide reach and your article make a huge impact on developers. I appreciate your work and dedication that you put to bring this in front of us all. Having said that I want to invite you to partner me in clearing the space and providing the correct Design patterns as they are and not as they occur to you, me or any other author. I want to point out that the example you have put is neither of that. In the factory pattern , the factory class has an abstract method to create the product and lets the sub classes to create the concrete product.
Use Cases of the Factory Method Design Pattern in Java
AccountType consists of fixed values that have been predefined in the banking industry domain; therefore, it is a constant, and we use Enum for this data type to ensure integrity. It's like a factory of factories which would, using the same approach, instantiate all spaceship-related factories with only a single new call at the start. In the next article, I will discuss the Real-time Examples of the Factory Design Pattern in C#. Here, in this article, I try to explain the Factory Design Pattern in C# with Examples. Please post your feedback, questions, or comments about this article. Unfortunately, they can also obscure the code and make it less readable.
For Spotify and Pandora, you register an instance of their corresponding builder, but for the local service, you just pass the function. It also requires a client key and secret, but it returns a consumer key and secret that should be used for other communications. As with Spotify, the authorization process is slow, and it should only be performed once. Imagine that the application wants to integrate with a service provided by Spotify. This service requires an authorization process where a client key and secret are provided for authorization. The biggest challenge to implement a general purpose Object Factory is that not all objects are created in the same way.
In this example, we’ll create a simple shape factory that produces different shapes (e.g., Circle and Square). Consider a software application that needs to handle the creation of various types of vehicles, such as Two Wheelers, Three Wheelers, and Four Wheelers. Each type of vehicle has its own specific properties and behaviors. In this article we'll describe a flavor of factory pattern commonly used nowdays. You can also check the original Factory Method pattern which is very similar. The Factory Method Pattern is one of several Creational Design Patterns we often use in Java.
The configuration is used as the keyword arguments to the factory regardless of the service you want to access. The factory creates the concrete implementation of the music service based on the specified key parameter. Another example in PHP follows, this time using interface implementations as opposed to subclassing (however, the same can be achieved through subclassing). It is important to note that the factory method can also be defined as public and called directly by the client code (in contrast with the Java example above). Each concrete class will implement this method to create a new object of the same type as itself. We also have to change the registration method such that we'll register concrete product objects instead of Class objects.
This operation doesn't require any change in the factory class code. The Factory Method defines a method, which should be used for creating objects instead of using a direct constructor call (new operator). Subclasses can override this method to change the class of objects that will be created. Then, using a SpaceshipFactory as our communication point with these, we'll instantiate objects of Spaceship type, though, implemented as concrete classes.
No comments:
Post a Comment